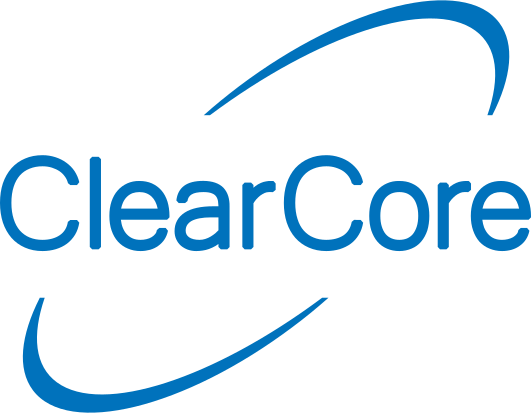 |
ClearCore Library
|
Loading...
Searching...
No Matches
23#ifndef HIDE_FROM_DOXYGEN
33#ifndef __ETHERNETAPI_H__
34#define __ETHERNETAPI_H__
39#define TX_BUFF_CNT (8)
43#define RX_BUFF_CNT (16)
47#define TX_BUFFER_SIZE (520)
51#define RX_BUFFER_SIZE (128)
132 GMAC_RX_DESC *rxDesc;
133 GMAC_TX_DESC *txDesc;
134 uint8_t *rxBuffIndex;
135 uint16_t *txBuffIndex;
139typedef struct netif netInt;
140typedef struct pbuf packetBuf;